Unlock Creative Power: Advanced Photo Editing & Side-by-Side Compare
When it comes to organizing digital life, ProShelf is already known for its beautiful blend of AI smarts and Mac-native design. But ProShelf is more than just a file organizer—it’s now also your creative playground, putting real photo editing muscle right inside your shelf. Let’s dive into the powerful new photo editing and effects capabilities, and shine a spotlight on our intuitive side-by-side compare feature.
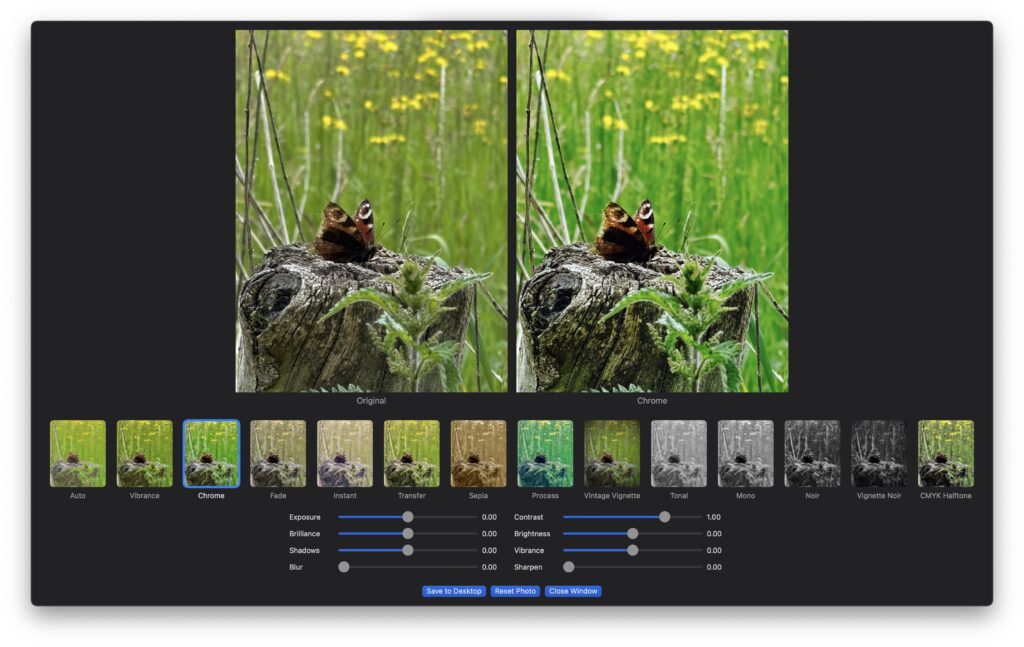
All-in-One Photo Editing, No Exports Needed
Tired of switching between apps to make simple edits? With ProShelf, you don’t have to. Open any image right in ProShelf, and instantly access a robust set of editing tools that cover everything from basic adjustments to artistic enhancements.
Key Features:
- One-Click Filters: Apply stunning looks and quick fixes with our curated set of customizable filters.
- Color Adjust & Tints: Fine-tune brightness, contrast, and color, or add a creative flair with tints.
- Undo / Redo Everything: Non-destructive edits with a full history make experimentation totally safe.
🎨 14 Professional Filters
- Auto Enhance – Intelligent one-click optimization
- Vintage Collection – Chrome, Fade, Instant, Transfer effects
- Artistic Styles – Sepia, Process, Tonal, Mono, Noir
- Creative Effects – Vintage Vignette, Vignette Noir, CMYK Halftone
- Color Enhancement – Dedicated Vibrance filter
⚡ Real-Time Adjustments
- Exposure Control – Perfect lighting in any condition
- Contrast & Brightness – Fine-tune your image’s mood
- Shadows & Brilliance – Professional highlight/shadow recovery
- Vibrance & Saturation – Make colors pop naturally
- Blur & Sharpen – Creative focus control
🔄 Transform Tools
- Rotation – 90° increments with smooth animation
- Mirror/Flip – Horizontal flipping for creative compositions
- Zoom Control – 0.5x to 4x magnification with precision slider
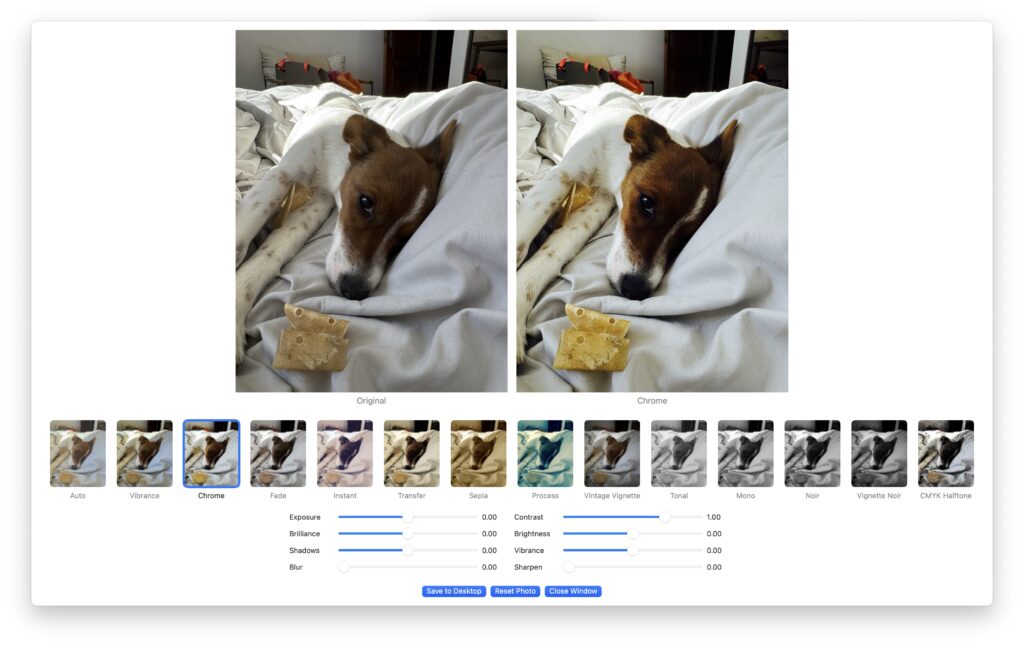
See the Difference: Side-by-Side Compare
One feature users are especially loving is Side-by-Side Compare. We know the uncertainty—sometimes you wonder, “Did my edit really improve the photo?” or “How does the enhanced version stack up against the original?” Now, with a click, you can see the transformation for yourself.
How It Works:
- Tap on a filter button or change a effects slider
- Instantly view your original image and the edited version side-by-side.
- Drag a slider (or swap left/right panels) to inspect details up close.
- Revert changes if you’re not satisfied—or export/share the improved version with confidence.
This feature isn’t just for perfectionists. It empowers everyone to make informed creative choices, experiment freely, and build trust in their editing workflow. See your improvements in real time, and never worry about “over-editing” or losing your unique vision.
Work Faster, Save Smarter
With ProShelf’s photo tools, you don’t need to juggle photo editors and file managers. Every adjustment is saved right where your photos live.
Ready for a Better Photo Experience?
If you want to edit, and compare photos as naturally as managing files, ProShelf is ready for you. Update today, and experience smart, delightful editing.